So I've been using
tmux for a few months now and here are some observations, a philosophy lesson, motivational speech and even some tips that might be useful.
At first you ask yourself, do I really need this?
Yes, use it. Like many tools that are powerful, the first few confusing encounters that expose the complexity of the tool and your ignorance of it, prevent the less persistent among us from sticking with it. Be persistent and dogged in your experiments with new tools that have the potential to make you more productive. Always.
My experience tells me that you need to stay with something for more than 10 minutes and work through the frustrations that are primarily a result of your lack of mastery and knowledge of the subject matter. Learn to read man pages. Exercise your patience. Stare at your microwave for the
entire 30 seconds as your coffee warms up! Embrace the meditative potential of the countdown toward your caffeinated nectar!
While sticking with hard things and being patient as you peel away your ignorance (paradoxically only to reveal more of it) seems obvious to those of us that have done
hard things in our life, it's a lesson many folks never embrace. Most find it easier to reach for the simpler, albeit less powerful implement, hence why there are so few master craftsmen in the world today. Instead we have Certified Charlatans (IT people with certifications out their butts, with no
real mastery of anything). Of course, the entire lesson of learning your tools well can applied to your approach to life. ;-)
Your fingers will be confused. It takes a while to get used to the key sequences
Get over it.
Ctrl-b is hard for me to reach too and confuses my already confused, arthritic, emacs trained fingers. Again, my ignorance of the tool shows, since customization is indeed possible and appears to be in my future. I'll probably suffer a bit more before heeding my own advice to master this tool.
Buddy Programming on Steroids
Pair or buddy programming is all the rage these days I guess. Technically I think pair programming requires a physical proximity, but maybe the term should be
Virtual Pair Programming or
Remote Pair Programming (RPP) or is that an oxymoron? Or is RPP like
Remote Viewing (RV) where we can use our psychic abilities to debug and troubleshoot? Cool!
Sharing a
tmux session and talking on Skype or Hangouts is infinitely better than IM alone and probably more effective that RV. I can't really imagine doing
remote buddy programming (RBP?) without using
tmux, Google Hangouts (screen sharing and audio) and the occasional IM message thrown in for good measure.
It works. In fact, I think r
emote buddy programming may be even more compelling and tolerable for some technical people who don't like the concept of close proximity with other humanoids. ;-)
I think the jury is still out on pair programming in general and taken to the eXtreme (pun intended) and forced on teams like
cod liver oil on children probably stinks as badly. Imposed on an unwilling group of programmers, PP will surely be as ineffective as
Han Solo, your supposed rock star developer who ignores personal hygiene for several days and emerges from the cave with the next
VisiCalc.
I like to throw those historical references out there for the kids.
Like all things in life,
it depends, and
your mileage may vary, for it is for the ends that you perform these actions, not merely the means themselves. As promised, a philosophy lesson, so let Aristotle explain...
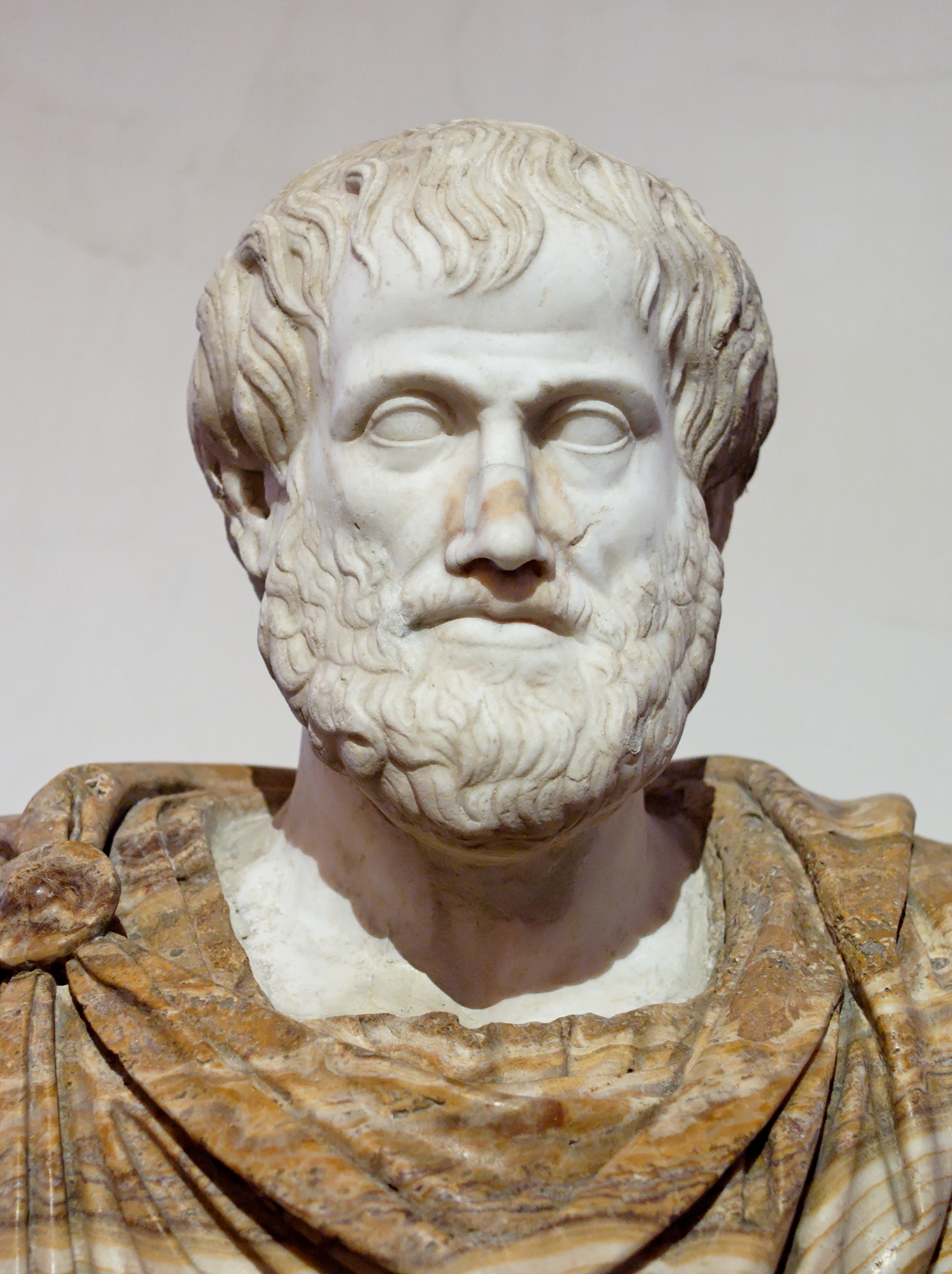
Every art and every inquiry, and similarly every action and pursuit, is thought to aim at some good; and for this reason the good has rightly been declared to be that at which all things aim. But a certain difference is found among ends; some are activities, others are products apart from the activities that produce them. Where there are ends apart from the actions, it is the nature of the products to be better than the activities. Now, as there are many actions, arts, and sciences, their ends also are many; the end of the medical art is health, that of shipbuilding a vessel, that of strategy victory, that of economics wealth. But where such arts fall under a single capacity- as bridle-making and the other arts concerned with the equipment of horses fall under the art of riding, and this and every military action under strategy, in the same way other arts fall under yet others- in all of these the ends of the master arts are to be preferred to all the subordinate ends; for it is for the sake of the former that the latter are pursued. It makes no difference whether the activities themselves are the ends of the actions, or something else apart from the activities, as in the case of the sciences just mentioned.
Savin' Your Bacon
The use case for
tmux if you have a spotty internet connection, or if you simply have to pick up and go because of some
non-maskable interrupt is quite compelling. I can't tell you how many times I've been happy to have my session pick up right where I left it after I was disconnected or told to take the trash out, which morphed into doing the dishes, walking the dog and oh by the way don't you dare go back to that computer!
Today's software development
modus operandi is develop
anywhere,
anytime. Starbucks (or Dunkin' Donuts), on an airplane with my
Chromebook and Gogo inflight access, in bed, by the TV, in my office, in my den, at the trade show making last minute changes, and even in my car on I-95 using a wifi hotspot and my Macbook while my wife gets lost going around Baltimore. If you want to open your Chromebook, boot in seconds (yup!), and fix a bug, then ssh to your server, attach to your
tmux session and you're ready to rock 'n roll. No blog is complete without a plug for the Chromebook and specifically the Acer C720 (stick with Chromebooks that use the
Intel Haswell architecture for now)...
love me some Chromebook!
Tips & Discoveries of the Clueless
This wasn't supposed to be a
tmux tutorial, but quickly:
Open a new tmux session
$ tmux -S /tmp/$USER new -s $USER
Attach to an existing session
$ tmux -S /tmp/$USER attach -t $USER
Detaching from a session
To detach from your system (not end the session), press
Ctrl-b d. To end the session, exit the shell by typing exit. The important point here is you want to detach from your session and keep it active, then reattach at some later time.
$ tmux -S /tmp/$USER attach -t $USER
Sharing Your Session
Create a group such as
developers and make sure each user you want to allow in your session is a member of the group. Modify the group of your session socket.
$ sudo groupadd developers
$ sudo usermod -a -G developers fred
$ sudo chgrp developers /tmp/rlauer
What No Scroll Buffer?
Yes, there is a scroll buffer however my ignorance regarding using
tmux is so deep that I didn't even know where to look. Google to the rescue! Ctrl-PgUp (or Ctrl-b [) puts you in scroll mode or what tmux calls copy-mode. Use PgUp, PgDn to move around the scroll buffer, 'q' to quit scroll mode.
Multiple Windows
As you become more comfortable with
tmux, you'll want to create multiple shells so you can perform parallel tasks (editing, building, monitoring logs, etc). Use
Ctrl-b % to create a window vertically and
Ctrl-b " to create windows horizontally. Use
Ctrl-b & to kill current window or type
exit to exit the shell.
Be aggressive in using new windows and it will increase your productivity. Go for it!
Changing the window size
To change the window size use
Ctrl-b left/right, but quickly.
tmux times out after the
Ctrl-b press fairly quickly so you need to pound the left and right arrows to adjust your window size.
More Info
$ man tmux
...or if you are in a session, Ctrl-b ? to see the key bindings.
Review
The least you to need to know to say that at least you know the least...
Ctrl-b ? => show key bindings
Ctrl-b d => detach session
Ctrl-b left/righ5/up/down => navigate to other window
Ctrl-b [ => scroll mode (then q to quit)
Ctrl-b PgUp => likewise
Ctrl-b % => split window vertically
Ctrl-b " => horizontally
What Else?